post というルーティングを追加します。
前項で参考にさせて頂いたこのページを参考にします。
難しいものは、まずは動作することが重要なので、このページの内容はわかりやすくて素晴らしいと思います。
前項で作成した vue-router プロジェクトをいろいろと変更します。
post を追加
サーバー起動して、http://localhost:8080 にアクセスします。
cd ~/vue-router
npm run serve
App.vue を変更。post を追加します。
~/vue-router/src/App.vue
<template>
<div id="app">
<nav>
<router-link to="/">Home</router-link> |
<router-link to="/about">About</router-link> |
<router-link to="/post">Post</router-link>
</nav>
<router-view/>
</div>
</template>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
}
nav {
padding: 30px;
}
nav a {
font-weight: bold;
color: #2c3e50;
}
nav a.router-link-exact-active {
color: #42b983;
}
</style>
必ずしも必要ないのですが、HelloWorld.vue が少しうるさいのでついでに変更します。
~/vue-router/src/components/HelloWorld.vue
<template>
<div class="hello">
<h1>{{ msg }}</h1>
</div>
</template>
<script>
export default {
name: 'HelloWorld',
props: {
msg: String
}
}
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
h3 {
margin: 40px 0 0;
}
a {
color: #42b983;
}
</style>
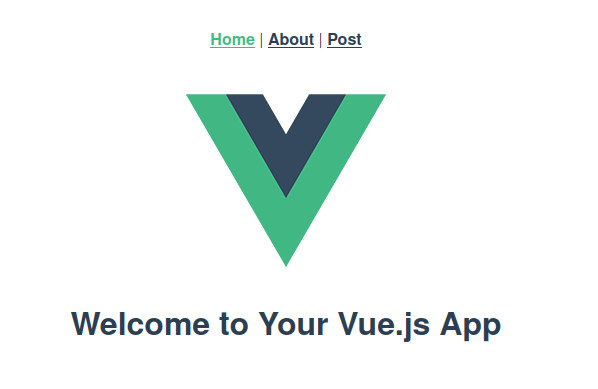
ルーティングの追加と vue ファイルの作成
index.js を編集します。
~/vue-router/src/router/index.js
import Vue from 'vue'
import VueRouter from 'vue-router'
import HomeView from '../views/HomeView.vue'
Vue.use(VueRouter)
const routes = [
{
path: '/',
name: 'home',
component: HomeView
},
{
path: '/about',
name: 'about',
component: () => import('../views/AboutView.vue')
},
{
path: '/post',
name: 'post',
component: () => import('../views/PostView.vue')
},
]
const router = new VueRouter({
mode: 'history',
base: process.env.BASE_URL,
routes
})
export default router
PostView.vue を作成します。
~/vue-router/src/views/PostView.vue
<template>
<div class="post">
<h1>This is a post page</h1>
</div>
</template>
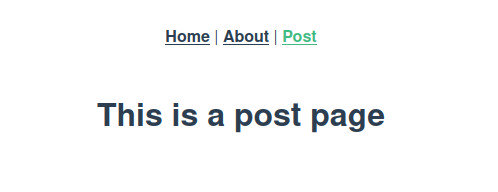
ここまでは何となく理解できます。
動的ルートの設定
PostView.vue を変更します。
~/vue-router/src/views/PostView.vue
<template>
<div class="post">
<h1>This is an Post page</h1>
<div v-for="post in posts" :key="post.id">
<router-link :to="`/post/${post.id}`">
{{ post.title }}
</router-link>
</div>
</div>
</template>
<script>
export default {
data(){
return {
posts: [
{
id:1,
title:'vue.js'
},
{
id:2,
title:'react'
},
{
id:3,
title:'alpine.js'
}
]
}
}
}
</script>
以下のようになります。
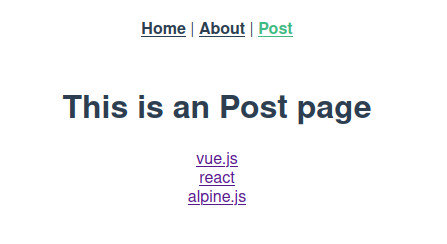
ルーティングの設定
~/vue-router/src/router/index.js
import Vue from 'vue'
import VueRouter from 'vue-router'
import HomeView from '../views/HomeView.vue'
Vue.use(VueRouter)
const routes = [
{
path: '/',
name: 'home',
component: HomeView
},
{
path: '/about',
name: 'about',
component: () => import('../views/AboutView.vue')
},
{
path: '/post',
name: 'post',
component: () => import('../views/PostView.vue')
},
{
path: '/post/:id',
name: 'PostShow',
component: () => import('../views/PostShow.vue'),
},
]
const router = new VueRouter({
mode: 'history',
base: process.env.BASE_URL,
routes
})
export default router
PostShow.vue を作成。
~/vue-router/src/views/PostShow.vue
<template>
<div class="post">
<h1>This is an Post Show page</h1>
</div>
</template>
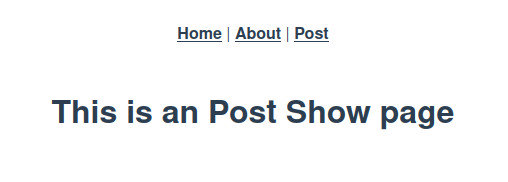
渡された変数を表示
PostShow.vue を変更します。
~/vue-router/src/views/PostShow.vue
<template>
<div class="post">
<h1>This is an Post Show page</h1>
<div>{{ $route.params.id }}</div>
</div>
</template>
id が渡っているのがわかります。
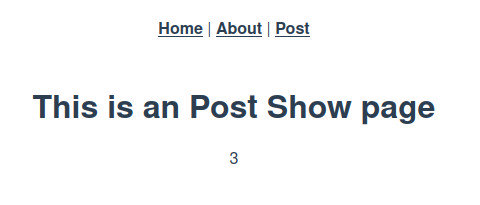
複数の変数を渡す
PostShow.vue を変更します。
~/vue-router/src/views/PostShow.vue
<template>
<div class="post">
<h1>This is an Post Show page</h1>
<div>{{ $route.params.id }}</div>
<div>{{ post.title }}</div>
</div>
</template>
<script>
export default {
data(){
return {
posts: [
{
id:1,
title:'vue.js'
},
{
id:2,
title:'react'
},
{
id:3,
title:'alpine.js'
}
]
}
},
computed:{
post(){
return this.posts.find(post => post.id == this.$route.params.id)
}
},
mounted(){
console.log(this.$route)
}
}
</script>
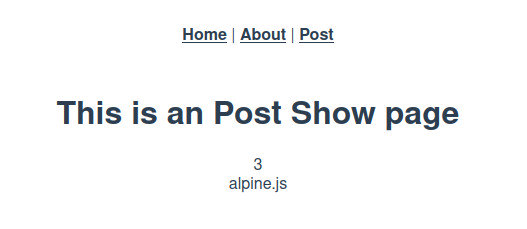
propsを利用してidを渡す
~/vue-router/src/router/index.js
import Vue from 'vue'
import VueRouter from 'vue-router'
import HomeView from '../views/HomeView.vue'
Vue.use(VueRouter)
const routes = [
{
path: '/',
name: 'home',
component: HomeView
},
{
path: '/about',
name: 'about',
component: () => import('../views/AboutView.vue')
},
{
path: '/post',
name: 'post',
component: () => import('../views/PostView.vue')
},
{
path: '/post/:id',
name: 'PostShow',
component: () => import('../views/PostShow.vue'),
props:true,
},
]
const router = new VueRouter({
mode: 'history',
base: process.env.BASE_URL,
routes
})
export default router
~/vue-router/src/views/PostShow.vue
<template>
<div class="post">
<h1>This is an Post Show page</h1>
<div>{{ post.id }}</div>
<div>{{ post.title }}</div>
</div>
</template>
<script>
export default {
props:{
id:{
type:String,
required:true,
}
},
data(){
return {
posts: [
{
id:1,
title:'vue.js'
},
{
id:2,
title:'react'
},
{
id:3,
title:'alpine.js'
}
]
}
},
computed:{
post(){
return this.posts.find(post => post.id == this.id)
}
},
mounted(){
console.log(this.$route)
}
}
</script>
参考サイトのコピーですが、私のような入門者にとっては大変に参考になるサイトです。
これを元にいろいろな実験をしてみようと思います。