これまでは同じ src にいくつもの js ファイルを平板的に置いてきましたが、Laravel でもそうだったように、ディレクトリ構成を直感的に理解しやすいようにして、それぞれのコンポーネントをインポートする方がいいようです。
ディレクトリ構成
今回は、まだ初心者なので、chatGPT の勧めに従って以下のようにしました。
~/my-routing-app/src
├── App.js
├── components
│ ├── About.js
│ ├── AnotherComponent.js
│ ├── NavLinkWithActiveStyle.js
│ ├── NotFound.js
│ ├── Post.js
│ └── Posts.js
├── index.js
├── pages
│ ├── Contact.js
│ └── Home.js
└── styles
├── App.css
└── index.css
App.js は下のように変更しました。
~/my-routing-app/src/App.js
import React from 'react';
import { BrowserRouter, Route, Routes } from 'react-router-dom';
import Home from './pages/Home';
import About from './components/About';
import Contact from './pages/Contact';
import Posts from './components/Posts';
import Post from './components/Post';
import NotFound from './components/NotFound';
import NavLinkWithActiveStyle from './components/NavLinkWithActiveStyle';
function App() {
return (
<BrowserRouter>
<header>
<h1>Hello React Router</h1>
<nav>
<ul>
{/* NavLinkWithActiveStyle コンポーネントの使用 */}
<li>
<NavLinkWithActiveStyle to="/">Home</NavLinkWithActiveStyle>
</li>
<li>
<NavLinkWithActiveStyle to="/about">About</NavLinkWithActiveStyle>
</li>
<li>
<NavLinkWithActiveStyle to="/contact">Contact</NavLinkWithActiveStyle>
</li>
<li>
<NavLinkWithActiveStyle to="/posts">Posts</NavLinkWithActiveStyle>
</li>
</ul>
</nav>
</header>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
<Route path="/contact" element={<Contact />} />
<Route path="/posts" element={<Posts />} />
<Route path="/posts/:id" element={<Post />} />
<Route path="*" element={<NotFound />} />
</Routes>
</BrowserRouter>
);
}
export default App;
他のコンポーネントのインポート
Laravel における blade のようなものがあるのではないかと思ったのですが、React では別々の js ファイルをインポートして使うようです。
例えば、以下のように About .js を変更します。
~/my-routing-app/src/components/About.js
import React from 'react';
import AnotherComponent from './AnotherComponent'; // 別のコンポーネントをインポートする
function About() {
return (
<div>
<h2>About Page</h2>
<p>This is About Page!</p>
<AnotherComponent /> {/* 別のコンポーネントを使用 */}
</div>
);
}
export default About;
つまり、AnotherComponent をインポートしてレンダリングします。
AnotherComponent は、
~/my-routing-app/src/components/AnotherComponent.js
import React from 'react';
function AnotherComponent() {
return (
<div>
<h2>AnotherComponent</h2>
<p>AnotherComponent</p>
</div>
);
}
export default AnotherComponent;
css を反映させる
React アプリケーションのエントリーポイントは、vueと同じく src/index.js のようです。
~/my-routing-app/src/index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import './styles/index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
reportWebVitals();
ここで index.css を読み込む設定になっています。
~/my-routing-app/src/style/index.css
body {
margin: 2em;
font-family: -apple-system, BlinkMacSystemFont, 'Segoe UI', 'Roboto', 'Oxygen',
'Ubuntu', 'Cantarell', 'Fira Sans', 'Droid Sans', 'Helvetica Neue',
sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
}
code {
font-family: source-code-pro, Menlo, Monaco, Consolas, 'Courier New',
monospace;
}
ul{
border:1px solid gray;
}
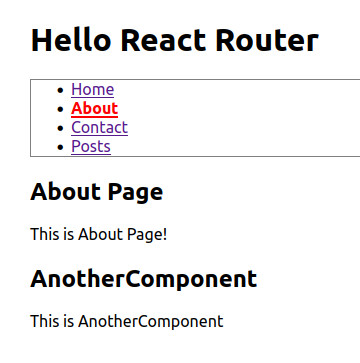
これはわかりやすい。Laravel よりわかりやすい気がします。
もっとも、こちらは front ですけどね。